Promise介绍
简单来说就是一个容器,里面保存着某个未来才会结束的时间(通常是一个异步操作的结果)
1 | const p = new Promise((resolve,reject) => {}) |
原型继承
所有的promise实例都具有这个方法
Promise.prototype.then()
1 | // fnc1 => resolve回调函数 func2 => reject回调函数 |
Promise.prototype.catch()
- 错误具有冒泡性质,所以catch可以捕获前面所有的错误
- catch也可以再抛出错误
1 | p.then(() => {}).catch((err) => {}) |
Promise.prototype.finally()
ES2018
1 | p.then().catch().finally() |
Promise.all()
传入Promise实例数组,不是promise实例,也会被promise.resolve()转换为promis实例 最后包装成一个新的实例
1 | const p = Promise.all([p1,p2,p3]) |
数组实例状态全为fulfilled,才为fulfilled,将数组的本身传给resolve回调 的参数以数组的方式传给all实例
数组中一个为rejected,则为rejected,第一个rejected参数传进
作为参数的promise有catch,则rejected时不会触发all的catch,而是将自身catch 后的参数添加进数组传给all
Promise.race()
跟随第一个状态改变
1 | const p = Promise.race([p1,p2,p3]) |
实例: 请求超时报错
1 | const p = Promise.race([ |
Promise.allSettled()
ES2020
1 | const p = Promise.allSettled([p1,p2,p3]) |
Promise.any()
ES2021
1 | const p = Promise.any([p1,p2,p3]]) |
1.至少一个为fulfilled,才为fulfilled
2.全为rejected,才为rejected
3.Promise.any()抛出的错误,不是一个一般的错误,而是一个 AggregateError 实例
Promise.resolve()
1 | 将现有对象转换为promise对象 |
注意点:立即resolve()的promise对象,是在本轮“事件循环”的结束时执行,而不是在下一轮“事件循环”的开始时
1 | setTimeout(function () { |
Promise.reject()
返回状态为rejected的promise实例
1 | 等同于new Promise(reject => reject()) |
Promise.try()
利用promise指定then下一步流程,同步函数同步执行,异步函数异步执行
(Promise里面的是可以同步函数同步执行,但是then和catch都是异步)
1 | 1.async方法: |
resolove(包括Promise.reolve())回调都是在本轮事件循环末尾时执行
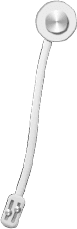
...
...
00:00
00:00
This is copyright.